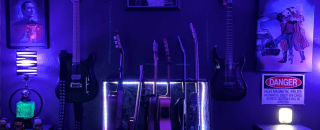
Appsmith supports writing JavaScript almost anywhere in the editor, like inside widget properties for text labels, colors, visibility and disabled state, etc. You can also import other JS libraries and use custom widgets to extend the platform even further. This is great for JavaScript devs, but what if you want to do something with Python? That's where our AWS Lambda integration comes in!
In this guide, we'll be building an initials icon generator to add those colored circles with user initials that you see in Gmail and other platforms. We'll use Python in an AWS Lambda function to generate an SVG that we can use in an app to enhance the UI.
This guide will cover:
- Creating/Deploying an AWS Lambda function with API Gateway
- Creating an Access Key in AWS
- Saving the Access Key as an Appsmith Lambda Datasource
- Triggering the Lambda function from Appsmith
Let's get started!
Creating/Deploying an AWS Lambda function with API Gateway
To get started, search Lambda in the AWS Console and select it from the search results.
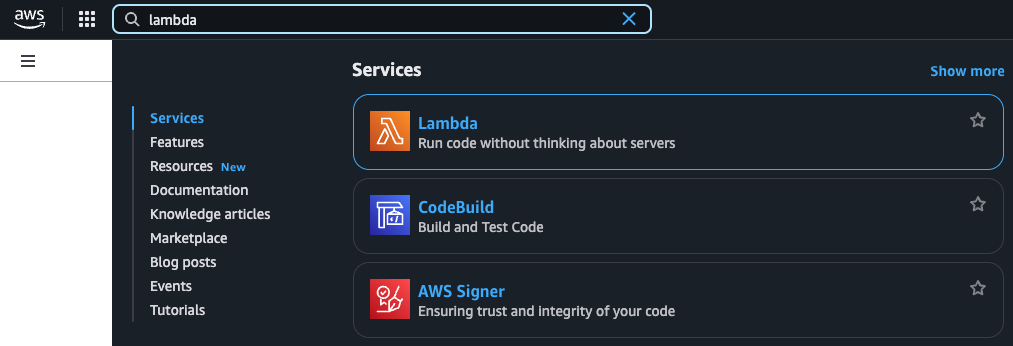
Then click Create function (author from scratch), give it a name, and chose the version of Python you'd like to use.
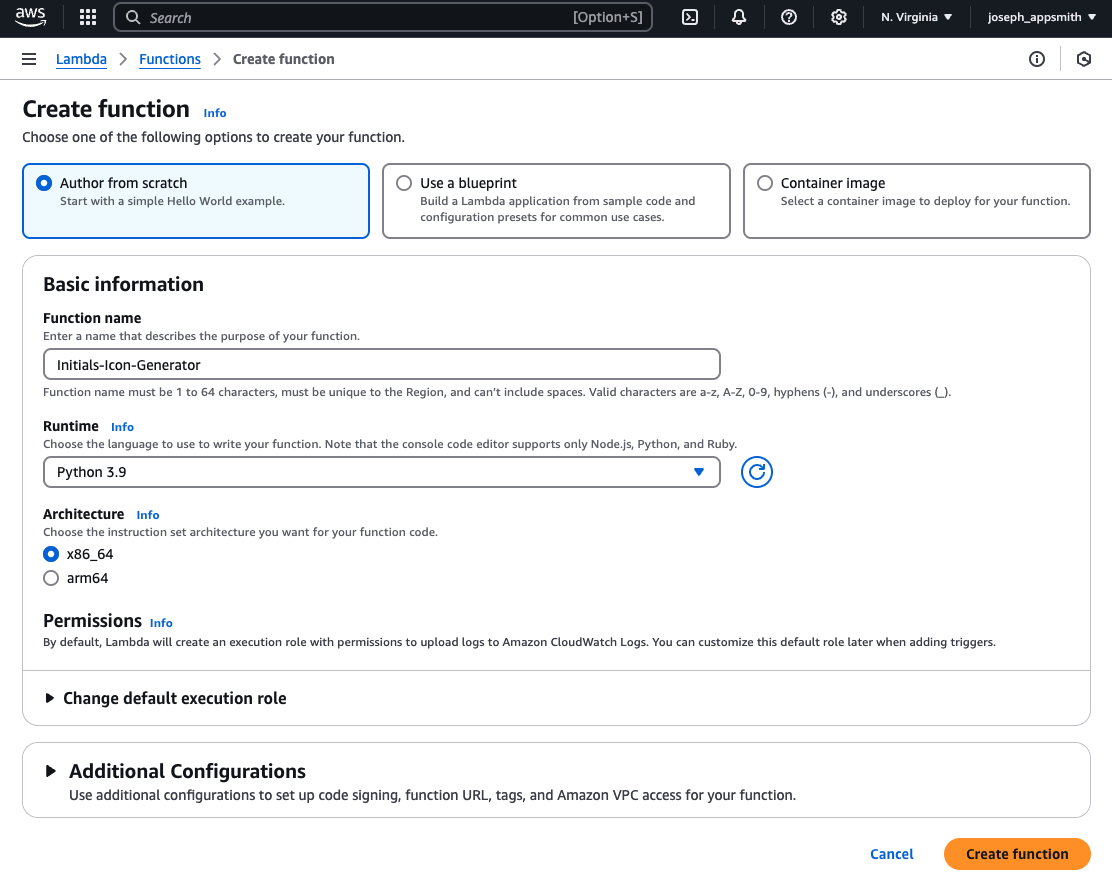
Click Create function to open the function editor.
Paste in the following code:
import base64
import hashlib
from urllib.parse import unquote_plus
def get_color(name):
hash_obj = hashlib.md5(name.encode())
hue = int(hash_obj.hexdigest()[:3], 16) % 360
return f"hsl({hue}, 70%, 50%)"
def create_svg(initials, color):
svg = f'''<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 100 100">
<circle cx="50" cy="50" r="45" fill="{color}"/>
<text x="50" y="50"
font-family="Arial"
font-size="40"
fill="white"
text-anchor="middle"
dominant-baseline="central">{initials}</text>
</svg>'''
return svg
def get_initials(fullname):
parts = fullname.strip().split()
initials = ''
for part in parts:
if part:
initials += part[0].upper()
return initials[:2]
def lambda_handler(event, context):
try:
# Get fullname from query parameters
query_params = event.get('queryStringParameters', {}) or {}
fullname = query_params.get('fullname', '')
# URL decode the fullname
fullname = unquote_plus(fullname)
if not fullname:
return {
'statusCode': 400,
'headers': {
'Content-Type': 'text/plain'
},
'body': 'fullname parameter is required'
}
initials = get_initials(fullname)
color = get_color(fullname)
svg = create_svg(initials, color)
return {
'statusCode': 200,
'headers': {
'Content-Type': 'image/svg+xml',
'Cache-Control': 'public, max-age=31536000',
'Access-Control-Allow-Origin': '*'
},
'body': svg
}
except Exception as e:
return {
'statusCode': 500,
'headers': {
'Content-Type': 'text/plain'
},
'body': str(e)
}
This will convert the fullname
string from the body into an SVG of the user's initials that we can use in Appsmith.
Next, click Add trigger (above code editor) search API Gateway, and select it.
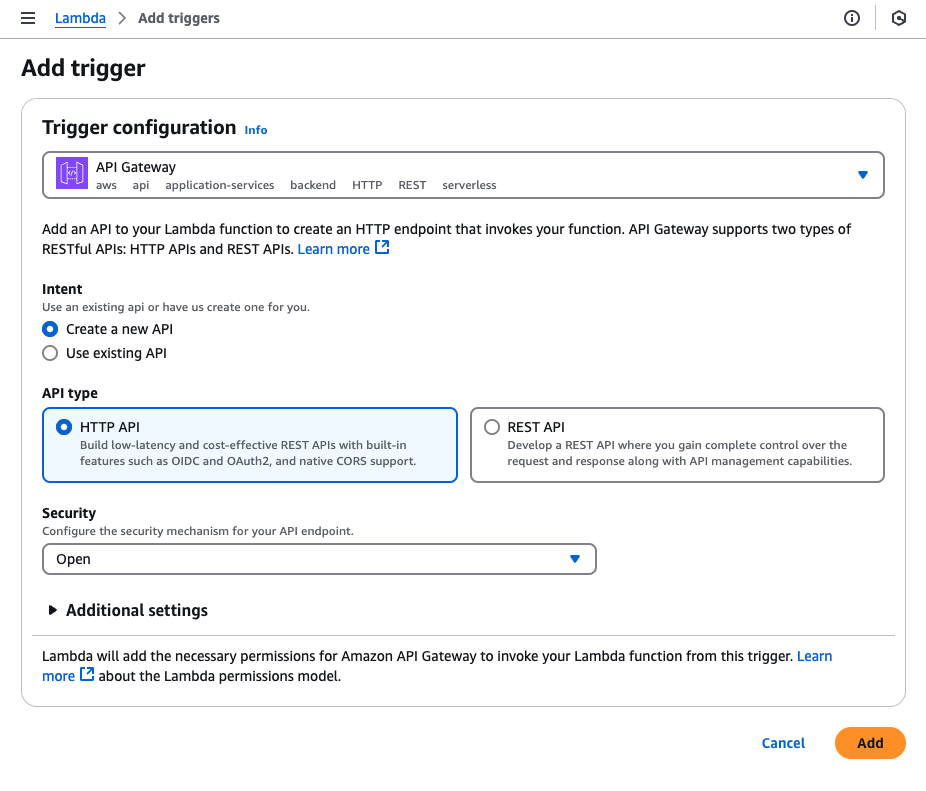
Notes:
- HTTP is sufficient for this use case, and cheaper than the full REST API.
- Security should be added, but that is outside the scope of this tutorial.
Click Add to save the new trigger. Then scroll back down to the editor, and click Deploy. The Lambda function can now be triggered from Appsmith's native integration, or from the endpoint url shown in the API Gateway configuration.
Creating an Access Key in AWS
Next, click Security & credentials from the User Profile menu (top right) and then click Create access key. This should be done from an IAM account, and not the root user.
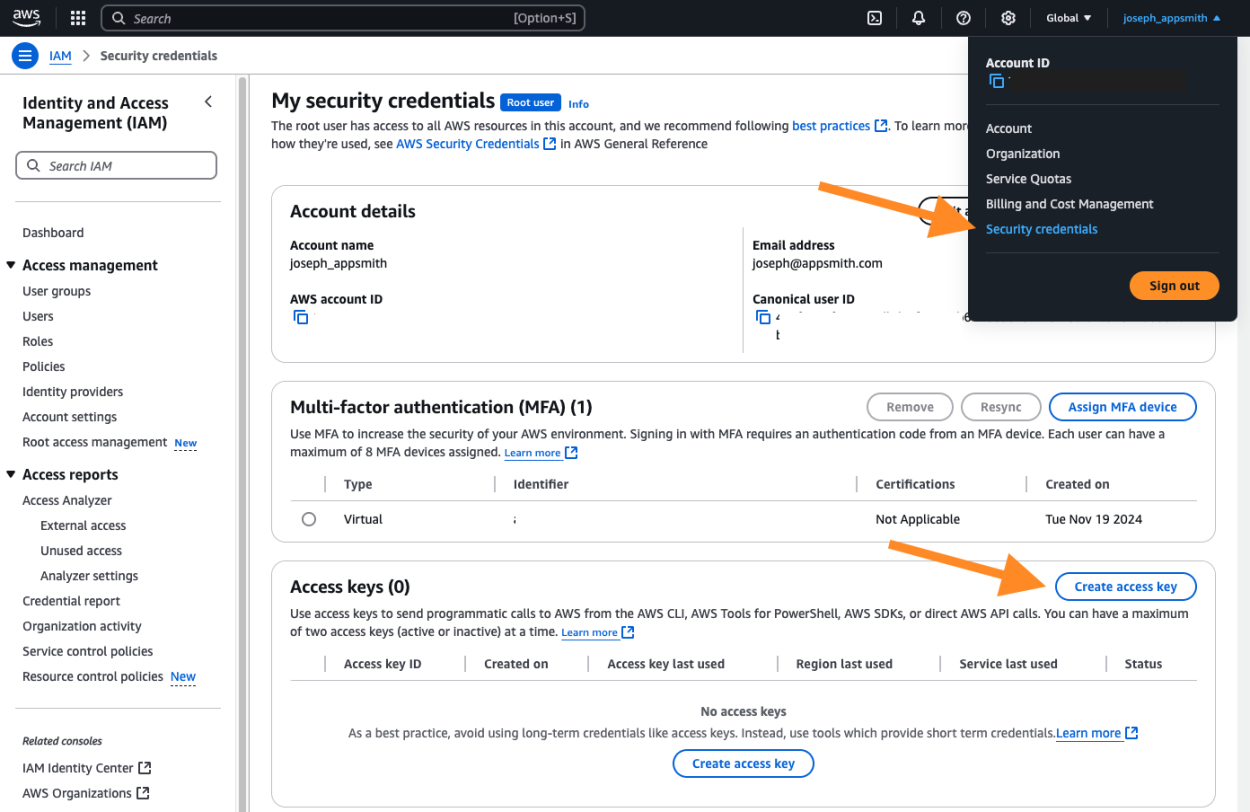
For the use case, choose Application running outside AWS, then click Next. Give the key a name, then click Create access key. Then leave this page open to copy the values in the next step.
Saving the Access Key as an Appsmith Lambda Datasource
Now open the app editor and click the Data icon on the left sidebar. Then click the + to add a new Datasource, and select Lambda.
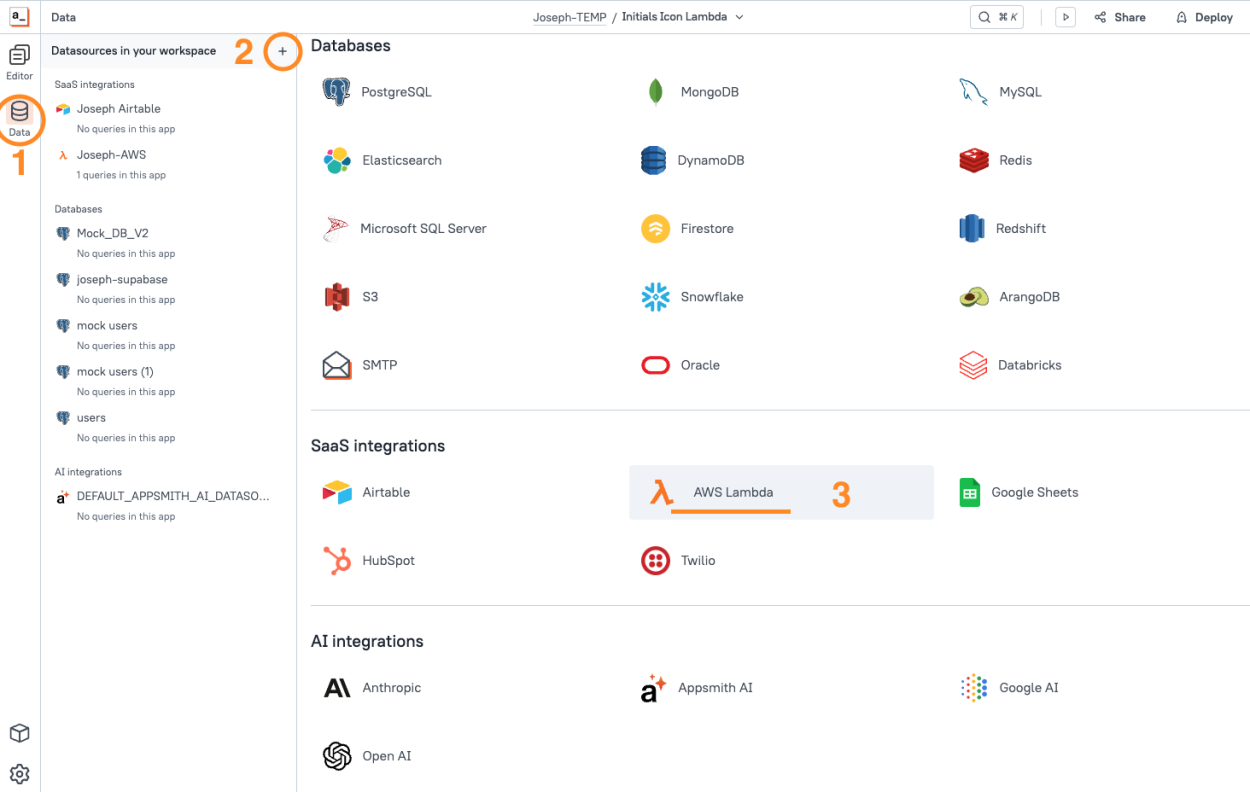
Name the datasource, then add your access key and secret and click Save.
Triggering the Lambda function from Appsmith
With the access key saved to an Appsmith datasource, you can now begin using the Lamdba function. Add a new API under the Lambda datasource, set the Command to Invoke a function, and choose your new Lambda function from the list.
Then set the body to:
{{
JSON.stringify({
"queryStringParameters": {
"fullname": "App Smith"
}
}
)
}}
Run the function and you should get back a response containing an SVG.
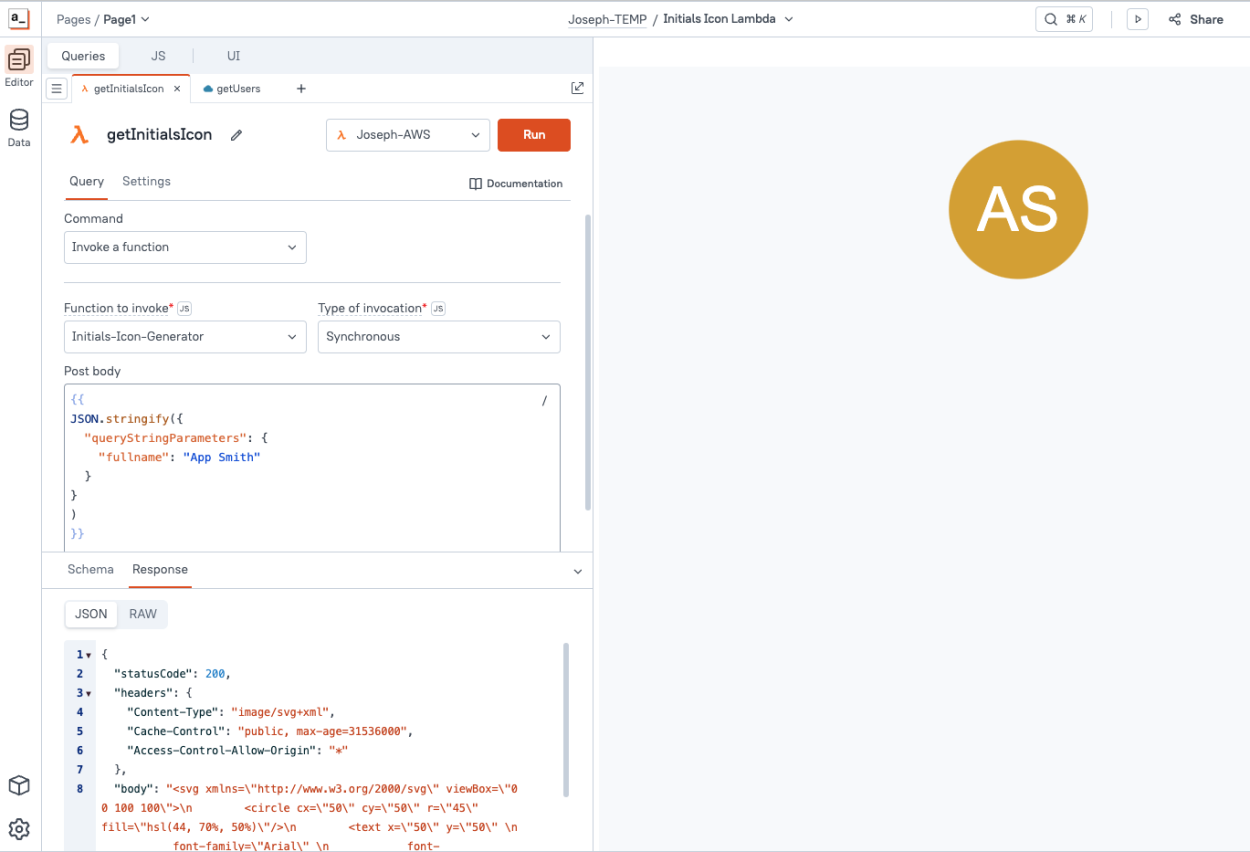
This can be displayed in an image widget by converting the raw SVG to a base64 dataUrl.
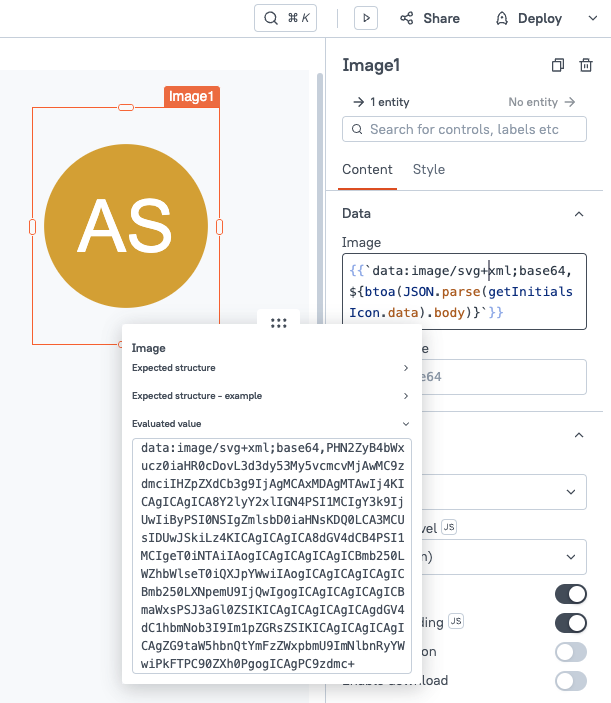
Displaying Initials Icon in a Table or List
The Appsmith Lambda integration can only return a single SVG at a time. But what if you want to display a list of users with all the initials icons at once? Just use the endpoint url from the API Gateway, and pass in the name as a url parameter.
{{`https://GATEWAY_ID.execute-api.us-east-1.amazonaws.com/default/Appsmith-Python-Demo?fullname=${currentRow.name.first} ${currentRow.name.last}`}}
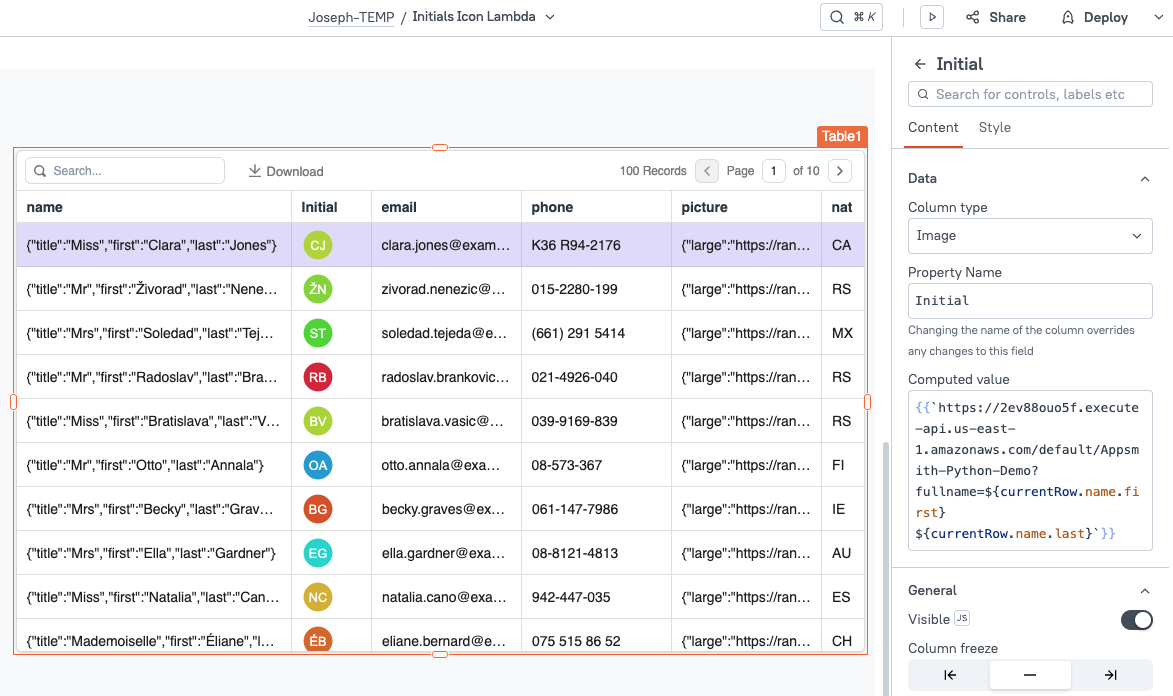
Conclusion
With Appsmith's AWS Lambda integration, you can easily extend Appsmith using Python code to transform data and enhance the UI. And for bulk usage in tables or lists, the endpoint url can be used with query parameters to add custom functionality.
What's Next?
This same technique could be used to generate colored tags or other graphics. Once you have your script set up, you could also save the SVG back to the user table and limit the number of function calls in AWS. Security should also be added to the API Gateway to prevent unintended usage.