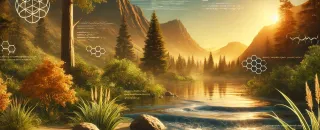
When building apps on top of data we typically want our UI to be reactive to changes in our data.
- Tables should updated when queries are ran
- Text fields should change based on the output of functions or other items
- Buttons should change their navigation depending on selections on the screen
One way to achieve this in Appsmith is by making use of the storeValue()
function. In this article we'll go over how to use it and a few common use cases we can solve with it.
How To Use The Appsmith Store
When we create a new app and enter a page for the first time, appsmith.store
is an empty object. We can add a new property with a particular key and value by calling storeValue('key', 'value')
. This function is async, so you'll need to await the call if you want to use the value in your code immediately after.
console.log(appsmith.store)
// {}
await storeValue('myKey', 42)
console.log(appsmith.store)
// { myKey: 42 }
await storeValue('myOtherKey', 'some value')
console.log(appsmith.store)
// { myKey: 42, myOtherKey: "some value" }
We can remove keys by passing the key name to the removeValue()
function.
await removeValue('myOtherKey')
console.log(appsmith.store)
// { myKey: 42 }
You can clear all keys in the store at once with clearStore()
.
await clearStore()
console.log(appsmith.store)
// {}
The storeValue()
function also takes a third parameter, persist
, that defaults to true. If you pass a false
value here then the stored value will be automatically cleared on navigation or page refresh.
console.log(appsmith.store)
// {}
await storeValue('myKey', 42, false)
console.log(appsmith.store)
// { myKey: 42 }
// After refreshing the page or navigating away
console.log(appsmith.store)
// {}
A note on storing Objects in the store:
It's possible to store JSON objects as keys in the store, and you can access them intuitively.
await storeValue('currentUser', { name: "Jim", favColor: "cyan" })
console.log(appsmith.store.currentUser.name)
// Jim
However, you cannot update keys of a stored object directly with storeValue('currentUser.name', 'John')
. Instead you must copy, modify, and overwrite the object like this.
let user = appsmith.store.currentUser
user.favColor = "red"
await storeValue('currentUser', user)
console.log(appsmith.store.currentUser.favColor)
// red
How Reactivity Works With Stored Values
Storing values helps us build reactive UI in Appsmith. Every time a stored value is updated all fields referencing them are also updated. Let's look at an example using the Table widget.
Table Data
Let's say we are running our fetchUsers
query, but we need to transform the response data for readability before displaying it in a Table widget. We can wrap the query in a function call that awaits the query, transforms the data, and stores the result. Let's create this fetchData
function in a new JS Object called JSObject1
:
export default {
async fetchData() {
const data = await fetchUsers.run()
// Transform the data
const transformedData = data.map(...)
// Store the transformed data in the store
await storeValue('userTableData', transformedData)
}
}
In our table we'll bind the data to the store with {{appsmith.store.userTableData || []}}
. Note the || []
at the end. This is to provide a fallback in case the function hasn't been called yet and the stored key is undefined
.
Now we can call JSObject1.fetchData()
when we want to run our query, and the table will automatically be updated with the transformed results. If this table need to be populated on page load you can enable this in the settings for JSObject1
.
Wrapping Up
By leveraging the Appsmith store, we can effortlessly build applications with reactive UIs that stay in sync with underlying data changes. In this article, we explored how to set, update, and clear values in the store using functions like storeValue(), removeValue(), and clearStore(). Whether you’re transforming data for a Table widget or dynamically updating UI components based on user interactions, these techniques help you maintain a single source of truth and reduce manual UI management.
Using stored values not only simplifies your code but also enhances your app’s responsiveness. As you build more complex applications, consider experimenting with persistent versus non-persistent values to suit your use case, and remember that every change in the store instantly propagates to your widgets. This reactive approach can be a game-changer in delivering a smooth, intuitive user experience.
If you don't have an account yet you can try us out completely free by signing up here. Happy coding!