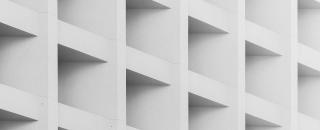
const getRandomNumber = (min, max, inclusive = false) => {
if (!inclusive) return Math.floor(Math.random() * (max - min) + min);
return Math.floor(Math.random() * (max - min + 1) + min);
};
Explanation:
There may be many cases in which we want to generate a random number and in JavaScript, we can do this by using the Math.random()
function.
Generating random numbers in JavaScript is not as intuitive as it is in other languages as the Math.random()
function only generates a floating-point number between 0 - 1. We often want to specify our own range and thus this snippet helps you do that.
By default, the
Math.random()
function is exclusive meaning that it includesthe lower bound number but excludes the upper bound number. For example, using
Math.random()
function will generate a floating-point number between 0 - 1but not including 1. Therefore, the number that is generated will never be 1.
The getRandomNumber
function mimics the Math.random()
function since by default getRandomNumber
is exclusive.
How to use the getRandomNumber Function
import getRandomNumber from "./getRandomNumber";
const min = 1;
const max = 500;
const includeMaxNumber = true;
const randomNumber = getRandomNumber(min, max, includeMaxNumber);
The getRandomNumber function has three parameters:
- A minimum value
- A maximum value
- An inclusivity parameter which is a boolean
I've added an inclusivity parameter that allows you to specify if you want to include or exclude the max number. By default this inclusivity parameter is set to **false**, therefore, it will act like the normal `Math.random()` function and generate a random number from the minimum value to the maximum value but not including the maximum value. By setting the inclusivity parameter to **true**, the `getRandomNumber` function will generate a random number from the minimum value to the maximum value and will include the maximum value.