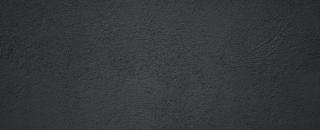
Create Self-Checkout System with the Code Scanner
Goal
By the end, you'll:
- Know how to effectively use a Code scanner widget.
- How to build a self-checkout system using the Code scanner widget.
Prerequisites
App connected to a datasource containing inventory information.
Overview
Configure query and function
Follow these steps to configure the query and functions:
- Drop a Code scanner and configure the Scanner layout property as required.
- Configure the query to retrieve product information based on the value obtained from the Code scanner:
SELECT * FROM inventory WHERE id = '{{CodeScanner1.value}}';
- Create a new JSOject and add a function to find the products:
export default { // Find function: Locates an item in the checkout array and returns its index, quantity, and the entire checkout array. find: (item) => { const checkout = appsmith.store.checkout || []; const i = checkout.findIndex(i => i.id == item.id); const q = checkout[i]?.quantity; return [i, q, checkout]; },... };
This function searches for a specific product in the checkout array, retrieving its index, quantity, and the entire array if found.
- Create new functions within the same JSObject to add and remove products to the checkout array:
// addToCheckout Function: Adds or increments the quantity of an item in the checkout array. addToCheckout: (item) => { const [i, q, checkout] = this.find(item); // If the item is found, increment its quantity in the checkout array. if (~i) { checkout[i] = { ...checkout[i], quantity: q + 1 }; } else { checkout.push({ ...item, quantity: 1 }); } // Update the checkout array in the store. storeValue('checkout', checkout); }, // removeFromCheckout Function: Decreases the quantity of an item in the checkout array or removes it if the quantity is 1. removeFromCheckout: (item) => { const [i, q, checkout] = this.find(item); if (q > 1) { checkout[i] = { ...checkout[i], quantity: q - 1 }; } else { checkout.splice(i, 1); } storeValue('checkout', checkout); },
In the above code
addToCheckout
function searches for the item in the checkout array and either increments its quantity or adds it with a quantity of 1.removeFromCheckout
function searches for the item in the checkout array, decrements its quantity if greater than 1, or removes it if the quantity is 1.- Add a new function to retrieve product information from the query:
getProduct: async ()=> { const [res] = await get_products.run(); this.addToCheckout(res); },
The above function asynchronously fetches product information and then adds the obtained product to the checkout array through the
addToCheckout
function.Display products
Follow these steps to display the products based on the values retrieved from the code scanner.
- Drop a Table widget and set the Table data property to:
{{appsmith.store.checkout}}
The
store
allows dynamic updates to the Table based on changes in the checkout array.- Add a new custom column in the Table, select the column type as an Icon button, and set its OnClick event to remove the item:
{{checkout_obj.removeFromCheckout(currentRow)}}
When triggered, this code removes the item associated with the current row in the Table from the checkout.
- Set the Code Scanner widget's OnCodeDetected event to run the
getProduct()
function:
{{checkout_obj.getProduct()}}
Conclusion
With this setup, whenever a valid barcode is scanned using the Code Scanner, the system retrieves product information through the query and adds the data to the Table. You can create additional functions to handle the checkout process according to your specific requirements.