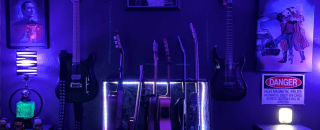
Unlike many other file upload UIs, Google Drive does not offer a way to upload a file from a URL. So when you have a file from a 3rd party that you want to save in drive, this means you have to download the file to your local machine first, just to turn around and upload it back to Google Drive. And if you have a lot of files to do, this can become a real pain.
If you've ever had to jump through these hoops, then this script is for you.
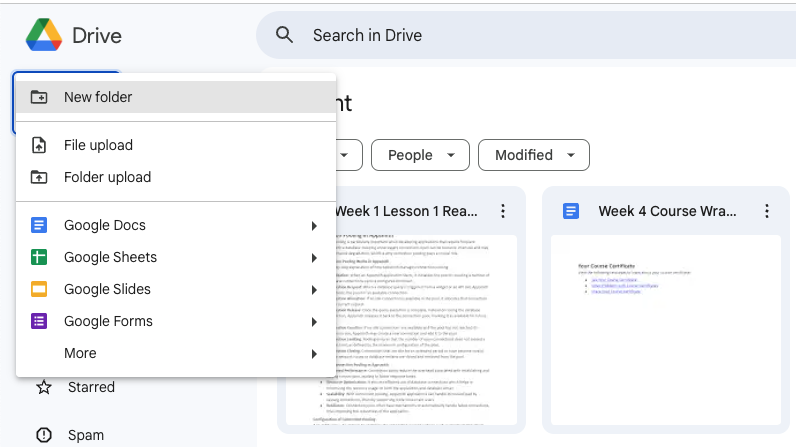
This script saves a file to Google Drive when you POST its URL to the webapp, and it returns the new URL for your copy of the file in Google Drive. Just send a POST request to the published script in this format:
{
'key': 'APIKEY',
'fileUrl': 'https://upload.wikimedia.org/wikipedia/commons/0/07/Reddit_icon.svg',
'folderId': 'FOLDER_ID'
}
The script checks the POST body for the API key, then saves the file to the specified folder in Google Drive. This can be used to extend other no-code/low-code tools, and provide a simple way to add images to Drive without the hassle of creating a google project, adding your credit card info, creating an API key, etc.
Just publish as a web app, and set the permissions to:
- Execute as: ME
- Who has access: ANYONE
const key = 'APIKEY'; // custom string to check in request body
const defaultFolder = 'FOLDER_ID_FROM_URL'; // folder to use if no id is given
const defaultUrl = 'https://upload.wikimedia.org/wikipedia/commons/0/07/Reddit_icon.svg';
function doPost(e) {
let returnedUrl = '';
let request = JSON.parse(e.postData.contents);
if (request.key == key && 'fileUrl' in request) {
returnedUrl = getFileByUrl(request.fileUrl, request.folderId);
}
return ContentService.createTextOutput(returnedUrl)
}
function getFileByUrl(url = defaultUrl, folderId = defaultFolder) {
// Download file from url and save to GDrive folder with fileName
const fileData = UrlFetchApp.fetch(url);
const folder = DriveApp.getFolderById(folderId);
const fileName = url.split('/').pop();
// string after last forwardslash: url/folder/filename.type
const newFileUrl = folder.createFile(fileData).setName(fileName).getUrl();
Logger.log(newFileUrl);
return newFileUrl;
}
NOTE: Make sure to run the script once to trigger the permission dialog, and click allow. Then publish the script as a webapp.
I've used this on several jobs to send files from other platforms to Google Drive. For added security, consider moving the API key to a script property instead of storing in in plain text in the script.
This is much easier and faster to set up than using the Google Drive API, and it's good enough for most use cases. Hope someone finds this helpful!
Decided to consolidate all of my old Google Apps Scripts from various tutorials and blog posts over the years. https://github.com/GreenFluxLLC/google-apps-script-utils