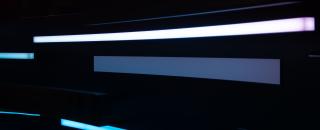
Have you ever been working with Javascript Objects and JSON, and wondered if they're just two names for the same thing? Well, you're not alone! But as it turns out, they have some key differences. Let's break it down in a way that's easy to grasp.
When you're learning how to code in Javascript, you'll soon bump into something called Javascript Objects. Think of them as backpacks where you can store the stuff you need for your code. Then, there's this thing called JSON, which stands for Javascript Object Notation. It's super popular for sending data around the web, like sending a text message from your phone. They look alike, but they have different jobs.
What's a Javascript Object?
JavaScript Objects are fundamental constructs used to store collections of data and more complex entities. They act as containers for named values (properties) and functions (methods when within objects), like a box where you can store things related to each other. These objects are super flexible, allowing for the encapsulation of data and functionality. In Appsmith, we use these as the primary way you create and manage the Javascript in your apps.
Note: The "proper" way to talk about object contents is properties and methods, instead of values and functions. While it doesn't matter too much, we will stick with the proper approach to keep things simple. Plus - it makes you sound like you know what you are talking about!
Defining Javascript Objects
JavaScript Objects can be defined using the object literal syntax, constructor functions, or ES6 classes. The properties are written as name:value
pairs (name and value separated by a colon) and enclosed by { }
(curly braces). Objects can also have methods, which are typically actions that can be performed on objects and are stored in properties as function definitions.
Here’s a simple example:
const cup = {
color: "black",
design: "cylindrical",
weight: 300,
material: "glass",
// Method to display the cup's description
getDescription() {
return `A ${this.color} ${this.material} cup, ${this.design} in design, weighing ${this.weight}g.`;
}
};
console.log(cup.getDescription()); // Outputs the description of the cup
This example illustrates the properties of a Javascript Object and how methods can be utilized to manipulate and interact with the object's data.
Introduction to JSON
JSON (Javascript Object Notation) is a way to write down information that computers and humans can understand. It's really good for sending data from one place to another, like from your computer to a website, because it's straightforward and easy to read. Its language-independent design draws on conventions familiar to programmers of the C-family of languages.
JSON Syntax and Structure
Although JSON resembles Javascript object literal syntax, it enforces stricter rules. Property names must be enclosed in double quotes, and they cannot contain comments or functions. JSON supports data structures like objects and arrays, making it versatile for a wide range of data representations. Here’s a basic JSON example:
{
"firstName": "Jane",
"lastName": "Doe",
"age": 28,
"isEmployed": true,
"address": {
"street": "456 Elm St",
"city": "Metropolis",
"zipCode": "67890"
},
"phoneNumbers": [
{"type": "mobile", "number": "123 456-7890"},
{"type": "office", "number": "987 654-3210"}
]
}
This JSON snippet demonstrates a structured representation of data about a person, including nested objects and arrays.
Key Comparisons Between Javascript Objects and JSON
Understanding the distinctions between Javascript Objects and JSON is important for knowing how and when to use them. Luckily, this isn't too hard..
Flexibility and Functionality
- Javascript Objects: They are highly flexible, capable of containing properties, methods, and supporting various data types, including
undefined
andDate
. This flexibility makes Javascript Objects suitable for building complex data structures and functionalities within the code. - JSON: Strictly a data format, JSON cannot hold methods. It is designed for data interchange between client and server or for configuration files. Its structure is simple and limited to text, making it easily parseable by various programming languages.
Syntax and Rules
- Javascript Objects: The syntax is more lenient, allowing unquoted property names, comments, and trailing commas. they can also contain symbols as keys and support methods.
- JSON: JSON follows a stricter syntax, requiring double-quoted property names, disallowing trailing commas, and excluding comments. This strictness ensures consistency and easy parsing across different systems and languages.
Usage and Application
- Javascript Objects: Primarily used within the code to organize and manipulate data, supporting complex data structures and functionalities.
- JSON: Serves as a language-independent format for transmitting data over a network, particularly in web applications. It is also used for configuration files due to its straightforward, text-based format.
Understanding Usage, Similarities, and Differences
When we talk about Javascript Objects and JSON, it's like discussing two different languages that share a lot of the same words. This can be confusing at first, but once you understand when and how to use each one, it all starts to make sense. Let's explore their usage, how they're similar and different, and why this might trip you up at first.
When to Use Each
- Javascript Objects: Imagine you're building a robot. Javascript Objects are like the internal wiring that makes the robot work. You use them when you're coding in Javascript to make your website or app do things. They're your go-to for storing data, organizing your code, and making your app interactive.
- JSON: Now, imagine your robot must send another robot a message. JSON is like the language they use to communicate. You use JSON when you need to send data from your website to a server or from one system to another. It's perfect for this job because it's easy for both humans and machines to understand.
How They're Similar
- Looks: Both Javascript Objects and JSON use a similar-looking structure with curly braces
{}
and key-value pairs. This makes them look almost identical if you're just glancing over code. - Data Representation: They both are great at representing complex data, like lists of information (arrays) or detailed records (objects within objects).
How They're Different
- Functionality: Javascript Objects can do more. They can have functions, which are bits of code that do something. JSON, on the other hand, is just information—no functions allowed.
- Syntax Rules: JSON is stricter. It requires double quotes around keys, and it doesn't allow comments or trailing commas. Javascript Objects are more flexible about these rules.
- Usage Context: Javascript Objects are used to make your app work. JSON is for talking between your app and other systems.
Why the Confusion?
The confusion between Javascript Objects and JSON usually comes down to their similarities in appearance and foundational concepts. Since JSON stands for Javascript Object Notation, it's easy to think of it as just another way to write Javascript Objects. But the key lies in their purpose and functionality. Think of it like this: all thumbs are fingers, but not all fingers are thumbs. Similarly, while JSON looks like Javascript Object notation, its capabilities and use cases are more specific.
Understanding this distinction is crucial. Imagine you're writing a letter (JSON) versus having a conversation (Javascript Objects). The letter is structured, follows specific formatting rules, and is meant to be understood by the recipient without further interaction. The conversation, however, can be more dynamic, with questions asked, answers given, and actions taken based on the discussion.
Practical Applications and Code Samples
To further demonstrate the differences, let’s explore practical applications of both Javascript Objects and JSON in web development scenarios.
Using Javascript Objects for Client-Side Logic
Javascript Objects are often used to structure and manipulate data on the client side. For instance, managing user input, controlling application state, or dynamically updating the UI based on user interactions.
// Example: Dynamically updating user profile information
const userProfile = {
name: "Alex Johnson",
age: 29,
updateProfile(name, age) {
this.name = name;
this.age = age;
// Code to update the UI
}
};
userProfile.updateProfile("Alexa Johnson", 30);
This example showcases how a Javascript Object can encapsulate both data and functionality, allowing for interactive and dynamic web applications.
Utilizing JSON for Server Communication
JSON is predominantly used for data interchange between a client and a server. It is the de facto standard for web APIs, enabling structured data exchange across different platforms.
// Example: Fetching user data from a server
fetch('https://api.example.com/user/12345')
.then(response => response.json()) // Parses the JSON response into a Javascript object
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In this example, JSON facilitates the exchange of user data between the web server and the client, highlighting its role in web APIs and server communication.
Conclusion
The distinctions between Javascript Objects and JSON, from their syntax to their usage in web development, are significant. Understanding these differences is essential for effective data manipulation and exchange in modern web applications. Through practical examples and detailed exploration, this article aims to provide a comprehensive understanding of these pivotal web development concepts, empowering developers to harness their full potential.
Is there a simple way to display the results of an appsmith query as json in a document, alongside a table widget, for example?
In reply to Is there a simple way to… by nilgai
Absolutely. If you bind the default query result to something like a Text widget, it is in JSON format already:
Just be sure to use
.data
and not.run()
. If you use.run()
, then it will actually make the DB query again instead of using the results you already retreived.In reply to Absolutely. If you bind the… by ron
Thanks for that Ron.
For the benefit of others, remember to use the Overflow text tab to constrain and scroll down a long list of json objects.
Also. to examine a selected table row as json displayed in a text widget, I had to save the selected row
from the table onRowselected option with
{{await storeValue('selectedRow', TblAlldocs.selectedRow)}}
and feed the Input widget with
{{appsmith.store.selectedRow}}
in its text box.I looked at displaying the json in an Input widget but it would only work as a fixed-size, unscrolable, multi-line text block;
a single-line text Input widget could only display the json as a realistically, unreadable long string.
In reply to Absolutely. If you bind the… by ron
Great tips John!