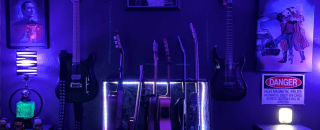
Introduction
JavaScript is a versatile language, allowing for different ways to define and execute code. Understanding key concepts like operators, expressions, functions, and Immediately Invoked Function Expressions (IIFEs) is essential for writing organized, performant JavaScript. In this post, we'll explore the definitions, syntax, characteristics and use cases of these core concepts, highlighting their distinctions. We'll also look at how they enable modular and reusable code.
Operators
Definition: Operators are symbols that perform operations on values and variables (operands).
Types:
- Arithmetic:
+, -, *, /, %, ++, --
- Assignment:
=, +=, -=, *=, /=
- Comparison:
==, ===, !=, >, <
- Logical:
&&, ||, !
- Conditional (Ternary):
condition ? expr1 : expr2
Characteristics:
- Used to perform calculations
- Enable evaluating conditions and logic flows
- Operators like
+=
and++
modify the existing variable
Operators are essential building blocks in JavaScript code. Learning how to properly leverage different operators allows creating complex logic while writing clean, efficient code.
For a complete list of operators check out the official Mozilla docs:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators
Expressions
Definition: An expression is a piece of code that evaluates to a value.
Types:
- Arithmetic:
3 + 4
- String:
"Hello " + "World"
- Logical:
true && false
- Assignment:
x = 10
An expression always evaluates to some value, which can be any valid JavaScript value including null, undefined, numbers, strings, objects, etc. The only exception to this rule is when an expression throws an error, which disrupts normal evaluation and prevents the expression from producing a value.
Functions
Definition: A function is a reusable block of code designed to perform a specific task.
Syntax: function functionName(parameters) { // Code to be executed }
Types:
- Named Functions:
function myFunc() {}
- Anonymous Functions:
const myFunc = function() {};
- Arrow Functions:
const myFunc = () => {};
Functions in JavaScript can be defined with, or without parameters, allowing them to accept inputs if needed. Functions may, or may not return a value. Each function has its own scope, meaning variables defined within a function are not accessible from outside the function, providing encapsulation and avoiding variable name conflicts.
IIFEs (Immediately Invoked Function Expressions)
Definition: An IIFE is a function that runs as soon as it is defined.
Syntax: (function() { // Code to be executed })();
In JavaScript, the use of parentheses around an Immediately Invoked Function Expression (IIFE) signals to the interpreter that the function is an expression, not a declaration. This allows it to be executed immediately. The parentheses following the function are the call to execute the function, ensuring it runs right away and establishes a private scope for its contents.
Using Concepts Together
These key JavaScript concepts often work together to create modular and reusable code:
Using Operators in Expressions
Operators enable complex expressions:
const x = 5;
const y = 10;
// Expression with multiple operators
const sum = x + x * y;
This leverages multiple arithmetic and assignment operators.
Using Expressions in Functions
Functions frequently leverage expressions to perform operations and return values:
function double(x) {
// Expressions:
const doubled = x * 2;
return doubled;
}
const result = double(2); // 4
Placing Functions in IIFEs
IIFEs can encapsulate functions to establish private scopes:
// IIFE creates private scope
(function() {
// Function is now scoped privately
function privateFunc() {
console.log('This function is private');
}
// Call the private function
privateFunc();
})();
This keeps privateFunc inaccessible globally.
As shown, these core concepts combine to allow efficient, modular JavaScript. Expressions bring computation power, functions and IIFEs enable encapsulation and reuse, and operators tie everything together. Understanding how they complement each other unlocks clean and scalable code.
Conclusion
Understanding the differences between expressions, functions, and IIFEs in JavaScript is crucial for writing efficient and organized code. Each has its unique use cases and understanding when to use them can significantly enhance your JavaScript coding skills.