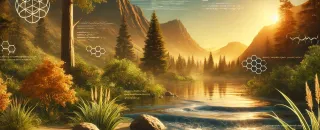
In JavaScript there are several ways to join two arrays. Some methods mutate the original array, while others create a new one. Each method offers unique features for joining arrays so you can choose the one that best fits your needs. Hereβs a breakdown of common approaches covering return values and mutability.
1. Using concat()
- Docs: MDN | Array.prototype.concat()
- Syntax:
const result = array1.concat(array2);
- Return Value: New array containing elements of both arrays.
- Mutates Original Arrays? No.
- Notes: A non-mutative, safe method compatible with older JS environments.
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
const combined = arr1.concat(arr2); // ["π", "π", "π", "π"]
// arr1 and arr2 remain unchanged
2. Using Spread Operator (...
)
- Docs: MDN | Spread syntax (
...
) - Syntax:
const result = [...array1, ...array2];
- Return Value: New array with elements from both arrays.
- Mutates Original Arrays? No.
- Notes: Concise and immutable. Ideal for modern JS.
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
const combined = [...arr1, ...arr2]; // ["π", "π", "π", "π"]
3. Using array1.push(...array2)
- Docs: MDN | Array.prototype.push()
- Syntax:
array1.push(...array2);
- Return Value: New length of the modified array (
array1
). - Mutates Original Arrays? Yes, modifies
array1
in place. - Notes: Simplified in-place mutation.
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
arr1.push(...arr2); // arr1 is now ["π", "π", "π", "π"]
4. Using unshift()
- Docs: MDN | Array.prototype.unshift()
- Syntax:
arr1.unshift(...arr2);
- Return Value: New length of the modified array (
array1
). - Mutates Original Arrays? Yes.
- Notes: This works just like
push()
but adds the elements to the start of the array.
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
arr1.unshift(...arr2); // arr1 is now ["π", "π", "π", "π"]
5. Using for
Loop
- Docs: MDN |
for
statement - Syntax:
for (let i = 0; i < array2.length; i++) {
array1.push(array2[i]);
}
- Return Value: None (directly modifies
array1
). - Mutates Original Arrays? Yes, modifies
array1
. - Notes: Offers compatibility and control, though more verbose than modern options. Good for combining and transforming arrays at the same time
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
for (let i = 0; i < arr2.length; i++) {
arr1.push(arr2[i]);
}
// arr1 is now ["π", "π", "π", "π"]
6. Using reduce()
- Docs: MDN | Array.prototype.reduce()
- Syntax:
const result = array2.reduce((acc, val) => acc.concat(val), array1.slice());
- Return Value: New array with elements of both arrays.
- Mutates Original Arrays? No.
- Notes: Creates a new array by reducing
array2
into a copy ofarray1
. Also good for combining and transforming arrays at the same time.
const arr1 = ["π", "π"];
const arr2 = ["π", "π"];
const combined = arr2.reduce((acc, val) => acc.concat(val), arr1.slice()); // ["π", "π", "π", "π"]
Summary
Now you should be armed with all of the information you need to choose the right method for your use-case. All of these methods are Baseline compatible which means they will work with Safari, Chrome, Edge, and Firefox browsers.
This is exactly what I need.Straight to the point with no filler to waste my time. A+
Looks good, thanks
The idea of using emojis as array elements is great!
Thanks for sharing this insightful post!