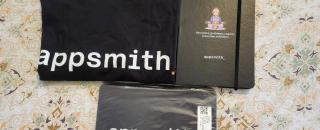
Text-To-Speech Synthesis Using Appsmith & Resemble.ai
Goal
Integrate Resemble.AI API with Appsmith & convert Text to speech
Prerequisites
Resemble.AI API Token
Appsmith Account
Overview
Integrating Resemble.AI API with Appsmith for Text-to-Speech Conversion. Resemble AI provides a powerful Text-to-Speech API, allowing you to generate realistic speech from text. You can integrate it into Appsmith to create a simple application where users enter text, and it gets converted into speech.
Get Resemble.AI API Key
- Sign up at Resemble AI.
- Navigate to API Settings and generate an API Key.
Create New Appsmith Application by Login to Appsmith Account
- Visit Appsmith Cloud to sign up or log in.
- Create a New Application and select a suitable theme.
- Add Form widget with Select widget to select Voice & Project
- Display different voice & project options from Resemble.AI and let users choose
-
Input widget to Add Text to Convert Speech
Creating Authenticating Data source in Appsmith
-
Select Data source as Authenticated REST API
Fill out the form as follows:
- URL:
https://app.resemble.ai
- Authentication Type: Bearer Token
- Add Header <Authorization> : <Your Bearer Token>
- Name datasource as "ResembleAPIs"
-
Click SAVE. Your Datasource is now connected and ready to use.
- Create API query to get all voice list available
- Method: GET
- URL:
https://app.resemble.ai/api/v2/voices?page=1&page_size=10
- Parameters: Page=>1, page_size=>10
- Name your API query "getVoice", save it, and test the connection.
- Create API query to get all Project list available
- Method: GET
- URL:
https://app.resemble.ai/api/v2/projects?page=1&page_size=10
- Parameters: Page=>1, page_size=>10
-
Name your API query "getProjects", save it, and test the connection.
-
Create Authenticated Datasource for another URL for Synthesise Text to Speech
Select Datasource as Authenticated REST API
Fill out the form as follows:
- URL:
https://f.cluster.resemble.ai
- Authentication Type: Bearer Token
- Add Header <Authorization> : <Your Bearer Token>
- Accept-Encoding: gzip
- Name datasource as "ResembleAISyntesise"
-
Click SAVE. Your Datasource is now connected and ready to use.
Create API query to Convert Text to Speech
- Method: POST
- URL:
https://f.cluster.resemble.ai/synthesize
- Body: JSON Format body
{
"voice_uuid": "{{Select1.selectedOptionValue}}",
"project_uuid": "{{Select2.selectedOptionValue}}",
"title": "{{Input1.text}}",
"data": "{{Input2.text}}",
"precision": "PCM_16",
"output_format": "mp3"
}
- Name query "TextToAudio" & save it
- URL:
Convert Text To Speech
- Bind data of API getVoice with Select1 widget: {{getVoice.data.items}}
- Bind data of API getProject with Select2 widget: {{getProject.data.items}}
- Add Text for converting to Speech in Input2 widget
-
Convert button call the API "TextToAudio"
{{TextToAudio.run().then(() => {
ConvertJSObject.decodeAudio();
showAlert('Speech generated Successfully', 'success');
JSObject1.toggleContainerVisibility();
});}}
- API response has a field 'audioBase64' which contains the Base64 encoded audio data
- need to decode it to Binary string & create URL
-
Write JSObject "ConvertJSObject" with following code for decoding Base64 Audio
export default { decodeAudio: () => { const base64Audio = TextToAudio.data.audio_content; const decodedAudio = atob(base64Audio); const byteArray = new Uint8Array(decodedAudio.length); for (let i = 0; i < decodedAudio.length; i++) { byteArray[i] = decodedAudio.charCodeAt(i); } const audioBlob = new Blob([byteArray], { type: 'audio/mpeg' }); const audioUrl = URL.createObjectURL(audioBlob); return audioUrl; } }
- Add Audio widget for Play/ Pause Audio (Converted Speech)
-
Bind the Audio widget to the response data
{{ConvertJSObject.decodeAudio.data}}
Conclusion
By following the above steps, you can successfully integrate Resemble.AI with Appsmith to enable text-to-speech conversion. Users can enter text, click "Convert," and the API will generate speech, which can then be played directly within Appsmith using the Audio widget.