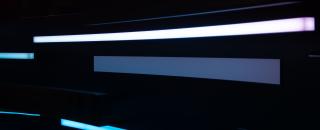
If you've looked at modern JavaScript code, you've probably seen some code that shows variables with three dots (...
), and you may have wondered what the heck that is. This versatile tool simplifies array and object manipulation, enabling developers to write cleaner, more efficient code. In this article, we'll delve into the spread operator, exploring what it is, how to use it, its best applications, as well as its pros and cons.
What is the Spread Operator?
The spread operator, denoted by ...
, allows you to unpack the elements of an array or the properties of an object into another array or object. This means you can take an array or object, and pass it as a group of items, rather than a single item.
In other words, it helps you take things from one array or object and put them into another array or object. It's like magic copying and pasting for programming! This seemingly simple syntax holds incredible potential, transforming the way we approach various coding scenarios.
How to Use the Spread Operator
Array Spreading
To spread the elements of one array into another, you simply use the spread operator followed by the array you want to spread.
const numbers = [1, 2, 3];
const moreNumbers = [...numbers, 4, 5];
console.log(moreNumbers); // Output: [1, 2, 3, 4, 5]
Object Spreading
For objects, the spread operator clones the properties of one object into a new one.
const person = { name: "Alice", age: 30 };
const updatedPerson = { ...person, age: 31, location: "Wonderland" };
console.log(updatedPerson); // Output: { name: "Alice", age: 31, location: "Wonderland" }
As you can see, the original array or object is spread out as a list of individual items that can be used to create a new array or object.
Alternatives to Using the Spread Operator
In order to understand the value of the spread operator, lets take a look at a few alternative options for doing the same thing. Here, we will look at a few ways to create or combine values into an array or object without using the spread operator.
Concatenating Arrays Without Spread Operator
This isn't too bad, and still has value. If you compare it to the example above, you can see its 2 lines of code instead of just two. In a simple case like this, the concat()
function is just as good. However, this can get problematic if you have more complex situations or the need to quickly spread the same data over multiple operations.
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = array1.concat(array2);
console.log(mergedArray); // Output: [1, 2, 3, 4, 5, 6]
Copying Object Properties Without Spread Operator
With objects, you can use Object.assign()
to get a similar result, and even in the same number of lines this time! However, if you look at this example compared to the first one, the first one is a bit cleaner and easier to understand (assuming you understand what the ...
means).
const originalObject = { name: "John", age: 25 };
const clonedObject = Object.assign({}, originalObject);
console.log(clonedObject); // Output: { name: "John", age: 25 }
In these examples, concat()
is used to merge arrays, and Object.assign()
is used to create a shallow copy of an object. While these alternatives work, they may require more lines of code compared to the concise spread operator.
Copying Object Properties Using a Loop
Many people (myself included) may initially think about using a loop to iterate over an object (or array), and then generate the new object from it. That is also a valid approach:
const originalObject = { name: "John", age: 25 };
const clonedObject = {};
for (let key in originalObject) {
if (originalObject.hasOwnProperty(key)) {
clonedObject[key] = originalObject[key];
}
}
console.log(clonedObject); // Output: { name: "John", age: 25 }
In this example, we are looping through the keys of the original object and copying each property to the clonedObject
. While this approach works, it's more verbose and error-prone than using the spread operator or Object.assign()
.
When to Use the Spread Operator
Merging Arrays
The spread operator simplifies the merging of arrays without modifying the original arrays.
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const mergedArray = [...array1, ...array2];
console.log(mergedArray); // Output: [1, 2, 3, 4, 5, 6]
Cloning Objects
Creating a shallow copy of an object is a breeze using the spread operator.
const originalObject = { name: "John", age: 25 };
const clonedObject = { ...originalObject };
Function Arguments
When calling a function that expects individual arguments, the spread operator lets you pass an array of values. This becomes even more powerful when you write your functions to expect a spread. When properly applied, this can reduce the need for code refactors in the future because your code is flexible.
function addNumbers(a, b, c) {
return a + b + c;
}
const numbers = [1, 2, 3];
console.log(addNumbers(...numbers)); // Output: 6
Pros of the Spread Operator
- Simplicity: The syntax is straightforward, making code more readable and concise.
- Immutability: The spread operator creates new objects/arrays, preserving the original data.
- Versatility: It works with arrays, objects, function arguments, and even iterables like strings and maps.
Cons of the Spread Operator
- Shallow Copy: While great for most use cases, the spread operator performs a shallow copy of nested objects, meaning deeply nested structures won't be cloned deeply.
- Performance: For very large arrays or objects, using the spread operator can impact performance due to memory consumption.
FAQ
Can the spread operator be used with other data types besides arrays and objects?
Yes! The spread operator can also be used with other iterable objects like strings, maps, and sets.
- When used with a string, it spreads each character of the string into individual elements.
- For maps and sets, it spreads each element into a new array or object.
How does the spread operator handle duplicate properties in objects?
When spreading properties from one object into another, if there are properties with the same name, the last property encountered in the spread operation will overwrite the earlier ones. This behavior allows for easy overriding of properties when combining objects but wasn't covered in the article. Understanding how duplicates are handled is crucial when merging objects to ensure the final object reflects the desired state.
What are the specific performance concerns with using the spread operator on large arrays or objects?
The spread operator can impact performance when used on very large arrays or objects due to increased memory consumption. This is because it creates a shallow copy of the elements or properties, which can be memory-intensive for large data structures.
Conclusion
Hopefully, at this point, you have a really solid understanding of what the spread operator is, how it works, and when to use it. Its ability to transform complex operations into elegant, easy-to-understand code is invaluable. Get familiar with the spread operator, and you'll empower yourself to write more efficient, readable, and maintainable code.