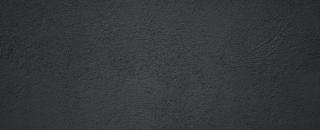
Baserow and Appsmith Integration Using JWT Tokens
Goal
By the end, you'll:
- Confidently integrate Baserow and Appsmith with JWT authentication.
- Effectively manage tokens for secure access.
- Execute Baserow APIs directly within Appsmith.
Prerequisites
Baserow Account: Sign up at https://baserow.io/.
Workspace and Database: Set up the workspace and database you'll use.
Basic Familiarity with REST APIs and JavaScript: This guide assumes some technical knowledge.
Overview
In the realm of application development, the synergy between these two powerful platforms can significantly enhance your capabilities. This step-by-step tutorial is designed to empower developers, allowing them to establish a robust connection between their Baserow database and the Appsmith framework.
In this tutorial, you'll learn the key techniques, such as setting up the authorization endpoint in Appsmith to generate tokens, creating a JavaScript object for efficient token management, and binding the JavaScript object to Baserow APIs.
Set Up Authorization Endpoint
The authorization endpoint validates users based on their Baserow email and password, allowing entry only to authorized individuals. Upon successful verification, the endpoint provides two essential tokens:
- Access Token: Your temporary passport for immediate entry, granting access to Baserow's APIs for a limited time.
- Refresh Token: Your renewal ticket, allowing you to obtain fresh access tokens when needed, keeping your connection active and secure.
To enable secure access and build a reliable connection between Appsmith and Baserow, follow these steps to set up the authorization endpoint.
- In Appsmith, create a new blank API in Appsmith named
api_authToken
- Set the method to POST and URL to
https://api.baserow.io/api/user/token-auth/
-
Provide your Baserow email and password in the body (JSON format).
{ "email": "your_baserow_email@example.com", "password": "your_secure_password" }
- Click Run to test the API and ensure it generates tokens successfully.
Create JS Object for Token Management
This JS Object will play a pivotal role in generating and storing tokens for seamless integration between Appsmith and Baserow. The Appsmith store will be used to store both the access and refresh tokens, along with the expiry information for the access token.
- Create a new JS Object named js_manage_tokens
-
Add code to:
- Generate access tokens
generateAccessToken() { //call the authorization api to generate a token const generatedToken = api_authToken.data; if (generatedToken) { //call the store method to add the generated token to the Appsmith store this.storeTokenInAppsmithStore(generatedToken.access_token, generatedToken.refresh_token); } }
- Store tokens and expiry in Appsmith's store
storeTokenInAppsmithStore(accessToken, refreshToken) { // add the access token, refresh token, and expiry of the access token in the store //using the storeValue() function storeValue("authAccessToken", accessToken); storeValue("authRefreshToken", refreshToken); storeValue("authAccessTokenExp", this.getTokenExpiry(accessToken)); }, getTokenExpiry(token) { try { return JSON.parse(atob(token.split('.')[1])).exp; } catch (error) { console.error('Error decoding token:', error); return null; } }
- Run the generateAccessToken() function to verify the response.
Add Refresh Token Endpoint
Create an additional blank API to interface with the Baserow refresh token endpoint. This refresh token API will handle the generation of tokens when an authentication token expires.
- Create a new blank API named
api_refreshAuthToken
- Set the method to POST and URL to
https://api.baserow.io/api/user/token-refresh/
-
Provide the refresh token from the store in the body (JSON format):
{ "refresh_token":{{appsmith.store.authRefreshToken}} }
- Click Run to test the API and ensure it refreshes tokens successfully.
- Create a new blank API named
Update JS Object for refresh endpoint
Let's add more capabilities to the JS object, like verifying the access token expiry, refreshing tokens, and a main method to handle over all logic for generating, validating, and refreshing tokens. Add the below code to the
js_manage_tokens
JS object.-
Verify token expiry
verifyAccessToken() { //verify the token expiry by accessing the token expiry date from the store and comparing it with the current timestamp return moment().utc() > moment().utc(appsmith.store.authAccessTokenExp); }
-
Refresh tokens
refreshAccessToken() { // call to const refreshToken = api_refreshAuthToken.data; if (refreshToken) { // update the Appsmith store with the new access and refresh tokens this.storeTokenInAppsmithStore(refreshToken.access_token, refreshToken.refresh_token); } else { console.error('Failed to refresh access token'); } }
-
Handle overall logic
getAccessToken() { // verify if the access token is present in the Appsmith store if (appsmith.store.authAccessToken) { // verify if the token expiry if (this.verifyAccessToken()) { //refresh the token this.refreshAccessToken(); } else { //generate an access token this.generateAccessToken(); } } // return the access token from Appsmith store return appsmith.store.authAccessToken; }
-
Bind JS Object to Baserow APIs
Now, you're ready to configure and execute Baserow APIs that require JWT authorization. Let's integrate the list_database_table_fields API designed to list all table fields. To do this, create a new API on Appsmith, configure the authorization header, and set the JWT access token from the Appsmith store as its value.
- Choose a Baserow API you want to execute. For example, list table fields.
- Create a new API in Appsmith and name it appropriately. For example,
api_listTableFields
. - Set the method and URL based on the Baserow API you chose.
-
For the authorization header, add:
Key: Authorization Value: JWT {{js_manage_tokens.getAccessToken.data}}
- This ensures your API call uses the valid access token secured by the JS object.
- Bind the newly created API to Table widget to visualize data.
Congratulations! Execute the requests, and you'll see your Baserow data seamlessly integrated into your Appsmith application. Securely leverage both platforms to build robust applications.
Bonus Tips
- Trailing Slashes for Baserow APIs: When working with Baserow APIs, ensure that your URLs end with a trailing slash. For example,
https://api.baserow.io/api/database/fields/table/
. Baserow expects valid URLs to have this trailing slash, and omitting it result in errors.
- Trailing Slashes for Baserow APIs: When working with Baserow APIs, ensure that your URLs end with a trailing slash. For example,
Conclusion
As we bring this tutorial to a close, you've navigated through the essential steps to establish a secure and streamlined connection between your Baserow database and Appsmith using JWT tokens. By following these guidelines, you're now ready to optimize your application development workflow. We encourage you to continue your learning journey and leverage these integration techniques to construct resilient and efficient applications.
Hi,
It's amazing, in part 5, can you give more example with screenshoot if possible. I have error 401 Unauthorized.
thanks