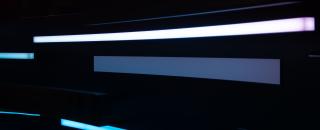
One of the key features of functional programming is recursion. A recursive function is one that calls itself. Wait - what? Yes - a function that loops over itself to do its thing. Why? Because we're adventurous like that! But also, because understanding recursion can open up a new perspective on problem-solving, and lead us to write elegant, self-explanatory, and surprisingly versatile code.
When Iteration Feels a Bit Loopy...
Let's begin with our familiar friend, the for
loop. It's been with us since we started learning to code, helping us count sheep when we can't sleep, and even graciously assisting us to traverse arrays and manipulate data.
But we've also had our fair share of messy break-ups (pun intended). It's not uncommon to find ourselves tangled in complex loop constructs, with several exit conditions and nested iterations, which could turn our code into a labyrinth of hard-to-navigate and debug logic.
Enter Recursion: The Self-Reflective Solution
In simplest terms, recursion is when a function calls itself until it doesn't. This technique is a fundamental concept in many programming languages and can sometimes offer a more elegant solution to problems that typically require loops.
function countDownFrom(n) {
console.log(n);
if (n > 0) {
countDownFrom(n - 1);
}
}
countDownFrom(5); // Outputs: 5 4 3 2 1
With recursion, our code becomes a straightforward translation of the problem's logic: start from number n
, print it, and then do the same for n - 1
. The base case (when n
is not greater than 0) stops the recursion.
Recursion Advantages
Recursion can make your code cleaner and easier to understand, especially when dealing with problems with a hierarchical or nested structure, such as traversing a file directory, manipulating nested arrays or objects, and solving complex mathematical problems. It can be a potent tool when used appropriately in your code:
- Clarity and Readability: Recursive functions can often be written in a more straightforward and readable way than their iterative counterparts. This makes the code easier to understand and maintain.
- Less Code: Recursive functions can frequently achieve the same result with less code than iterative solutions. This is particularly true when dealing with inherently recursive problems, like traversing tree-like data structures.
- Elegant Problem Solving: Problems involving complex nested structures, backtracking, or divide-and-conquer techniques often have elegant and intuitive solutions when approached with recursion.
- No State Management: With recursive functions, you don't need to keep track of the state with additional variables as you would in a loop. Each function call has its execution context, reducing the chance of bugs caused by mutable shared state.
- Natural Fit for Certain Data Structures: Recursive algorithms are a natural fit for certain types of data structures, such as trees and graphs. Functions to traverse or search these structures are often simpler and more intuitive when written recursively.
- Sequential Processing: In certain scenarios where you must ensure a sequence of operations happen one after another (for instance, when dealing with asynchronous tasks), recursion can help manage these sequential processes more intuitively than loops.
- Solving Complex Problems: Recursion is often used in algorithmic problems such as sorting, searching, and traversing. Algorithms like Merge Sort, Quick Sort, Tree, and Graph traversals are easier to implement with recursion.
Loops or Recursion?
Before we get deeper, let's understand when NOT to use recursion. Loops and recursion both have their strengths and weaknesses, and deciding when to use one over the other largely depends on the specific problem at hand and the context. Loops are generally more efficient in terms of performance, and they should be your go-to choice for simple iterations and manipulations on flat data structures. Recursion shines in scenarios where the problem is recursive, such as traversing a DOM tree or a file directory.
Here are some scenarios where using loops might be a more suitable choice:
- Performance Concerns: Loops are generally more efficient than recursion regarding time and space complexity. Recursive calls can lead to increased memory usage because each function call is added to the stack, while a loop only requires a single memory allocation. Using a loop may be a better choice if you're dealing with a large data set or performance-critical application.
- Language Limitations: Some languages, including JavaScript, have a maximum call stack size, which limits the depth of your recursion. You'll run into a stack overflow error if you exceed this limit. So a loop might be a safer option for problems where you expect deep recursive calls.
- Simple Iteration: If you're working with a flat structure (like a simple array or list) and performing a straightforward operation that doesn't involve nested elements or dependencies between elements, a loop is a more straightforward and efficient choice.
- Mutative Operations: If you're performing an operation that requires changing the state of a variable during each iteration, a loop is usually a clearer and more efficient solution.
- Unpredictable Termination: If the termination condition is not straightforward or predictable, using a loop with a clearly defined exit condition might be better.
- Understanding and Readability: If your team or collaborators are more comfortable with iterative constructs and could find recursive solutions confusing, it may be best to stick with loops.
As you can see, performance is the key issue to keep in mid. We must be cautious when using recursion in JavaScript due to the language's call stack limit. Exceeding this limit will result in a "Maximum call stack size exceeded" error. This can be mitigated by using techniques like tail call optimization (in ECMAScript 2015).
Remember, though, recursion isn't a magic bullet and doesn't come without its trade-offs. Excessive or inappropriate use of recursion can lead to issues like stack overflow errors and can be more computationally expensive than iterative solutions. Always weigh your options and use the right tool for the job.
More Recursive Fun!
Ok - so we have some ideas on when to use recursion, and when not to use it. Now, lets look at a few key examples to understand how this crazy recursion thing works.
1. Fibonacci Sequence:
The Fibonacci sequence is a classic recursion example. Each number in the sequence is the sum of the two preceding ones.
function fibonacci(n) {
if (n <= 1) {
return n;
} else {
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
console.log(fibonacci(10)); // Outputs: 55
2. Sum of an Array:
Although this is more efficiently done with a loop, it is a good recursion example.
function sumOfArray(array) {
if (array.length === 0) {
return 0;
} else {
return array[0] + sumOfArray(array.slice(1));
}
}
console.log(sumOfArray([1, 2, 3, 4, 5])); // Outputs: 15
3. Flatten a Nested Array:
This function recursively flattens nested arrays into a single array.
function flattenArray(array) {
if (array.length === 0) {
return [];
}
if (Array.isArray(array[0])) {
return flattenArray(array[0]).concat(flattenArray(array.slice(1)));
} else {
return [array[0]].concat(flattenArray(array.slice(1)));
}
}
console.log(flattenArray([1, [2, [3, 4], 5]])); // Outputs: [1, 2, 3, 4, 5]
4. Find a key in a nested object:
When you have a complex nested objects with a potentially unknown number of levels, this can be a great way to find a needle in a haystack.
function findKey(obj, key) {
if (key in obj) {
return obj[key];
}
for (let i in obj) {
if (typeof obj[i] === 'object') {
let found = findKey(obj[i], key);
if (found) return found;
}
}
return null;
}
FAQ
What's the Big Deal with Recursion and Loops?
When coding in JavaScript, you often encounter problems that need you to do something over and over, like going through a list of items. You can solve these problems using loops, like the for
loop, which is pretty straightforward. But there's another cool way called recursion, where a function calls itself to solve a problem bit by bit.
How Do I Choose Between Recursion and Loops?
Choosing between recursion and loops depends on what you're trying to do. If your task involves a lot of straightforward, repeat actions on things like lists or arrays, loops are your best friend because they're simple and don't eat up too much memory. Recursion is awesome for more complex stuff, like when you're dealing with nested lists or structures (think of a folder within a folder), because it can make your code cleaner and easier to follow.
What's This Tail Call Optimization Thing?
Tail Call Optimization (TCO) is a fancy term for a way that some programming languages, including JavaScript, can make recursion more memory-efficient. It's supposed to prevent your program from crashing by using too much memory if you're doing a lot of recursions. However, not all browsers and JavaScript environments handle it the same way, so it's a bit hit or miss whether you can rely on it to save your recursive functions from causing problems.
Recursion Sounds Cool, But How Do I Keep It From Getting Too Confusing?
Recursion can get tricky, especially when trying to figure out why something's not working right. When you're debugging (that's coder talk for fixing bugs in your code) recursive functions, start by checking your base case (the condition that stops the recursion) to make sure it's set up correctly. Also, take it slow and maybe use some code tracing tools or good old-fashioned pen and paper to keep track of what your function is doing at each step. It's like solving a mystery, and every little clue helps!
So, When Should I Really Use Recursion Over Loops?
Recursion is great for problems where you're dealing with things that have a lot of layers, like a tree or a set of nested folders. It makes your code look cleaner and can be easier to understand once you get the hang of it. Loops, on the other hand, are better for when you're just going through a list or array and doing something with each item. They're also a bit easier on your computer's memory.
Conclusion
Recursion is a powerful tool in your JavaScript toolbox. It can make your code cleaner, more elegant, and easier to understand. It can be very helpful in breaking down complex problems into simpler, smaller problems, and these examples are just the tip of the iceberg. However, use it wisely! Always consider the problem you're solving and the structure of your data.